Power BI is a powerful business intelligence tool developed by Microsoft, while Laravel is a popular PHP web application framework. Although they are different technologies, it is possible to integrate them to create a robust data analytics solution. There are a few ways to implement Power BI in Laravel Web Application, here are some possible approaches:
- Embedding Power BI Reports: Power BI provides an embed feature that allows you to embed Power BI reports in your web application. You can use the Power BI Embed SDK to achieve this. You can embed the report using an iframe or by using the JavaScript API to create a custom solution. You can then use Laravel to handle authentication and authorization for the embedded report.
- Using Power BI REST API: You can use the Power BI REST API to programmatically create, update and manage Power BI resources such as reports, dashboards, and datasets. You can use Laravel to build a backend API that interacts with the Power BI REST API. You can then use a front-end JavaScript framework to consume the API and display the data.
- Power BI and Azure: Azure is Microsoft’s cloud computing platform. You can use Azure to host your Laravel application and integrate it with Power BI. You can use Azure Active Directory to authenticate users and manage access to Power BI resources. You can also use Azure Functions to create custom APIs that interact with Power BI.
Implementing Power BI reports in a Laravel application using embedding can be done in a few steps. Here’s an overview of the process:
- First, you need to create a Power BI account and upload the report you want to embed in your Laravel application.
- Next, you need to register your application with Microsoft Azure Active Directory and create a new app registration. This will give you an app ID and a client secret that you’ll need to use later in the process.
- Once you have your app registration set up, you can use the Power BI PHP Client library to interact with the Power BI REST API. Install this library using Composer.
- Use the PHP client library to authenticate your application with Azure Active Directory, and then retrieve an access token that you can use to access your Power BI report.
- Use the access token to generate an embed token for your report. This will give your Laravel application permission to display the report.
- Finally, embed the report in your Laravel application using the Power BI JavaScript API.
Here are some more detailed steps to help you get started:
- Create a Power BI account and upload your report
- Go to https://powerbi.microsoft.com/ and sign up for an account if you don’t already have one.
- Upload the report you want to embed in your Laravel application to your Power BI workspace.
- Register your Laravel application with Azure Active Directory
- Go to the Azure Portal (https://portal.azure.com/) and sign in with your Microsoft account.
- Create a new app registration by navigating to Azure Active Directory -> App registrations -> New registration.
- Give your app a name and select “Web” as the application type.
- In the “Redirect URIs” section, add the URL where you’ll be embedding your Power BI report (e.g. https://localhost:8000/report).
- Click “Register” to create the app registration.
- Note the “Application (client) ID” and “Client secret” values, as you’ll need these later.
- Install the Power BI PHP Client library
- In your Laravel application, open a terminal window and navigate to your project directory.
- Run the following command to install the Power BI PHP Client library:
composer require microsoft/power-bi-php
- This will install the library and its dependencies.
- Authenticate with Azure Active Directory and retrieve an access token
- In your Laravel application, create a new controller method that will handle the authentication and token retrieval process.
- Use the following code as a starting point, replacing the placeholder values with your own:
use Microsoft\PowerBI\Auth\AuthenticationContext;
use Microsoft\PowerBI\Auth\Token;
use Microsoft\PowerBI\Enums\AuthorityType;
public function authenticate()
{
$authorityUrl = 'https://login.microsoftonline.com/common';
$clientId = 'your_client_id_here';
$clientSecret = 'your_client_secret_here';
$resourceUrl = 'https://analysis.windows.net/powerbi/api';
$username = 'your_power_bi_username_here';
$password = 'your_power_bi_password_here';
$authenticationContext = new AuthenticationContext($authorityUrl, AuthorityType::AAD);
$authenticationResult = $authenticationContext->acquireTokenForClient($resourceUrl, $clientId, $clientSecret);
$accessToken = $authenticationResult->getAccessToken();
return view('report', ['access_token' => $accessToken]);
}
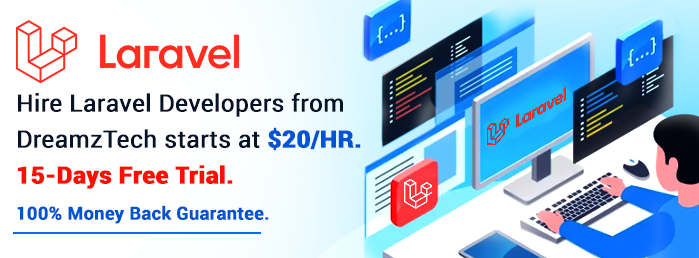
- This code creates an
AuthenticationContext
object, and then uses it to retrieve an access token using your app ID and client secret. - The access token is then passed to the
report
view, where it will be used to generate
Implementing Power BI reports in a Laravel application using the Power BI REST API can be done in a few steps. Here’s an overview of the process:
- Register an Azure AD application: To use the Power BI REST API, you need to register an Azure AD application and generate a client ID and secret key. Follow the steps in the Azure portal to create a new Azure AD application.
- Grant permissions: You need to grant permissions to the Azure AD application to access the Power BI API. Go to the Azure portal and select the Azure AD application that you created. Navigate to the “API permissions” tab and add the “Power BI Service” API. Select the appropriate permissions for your application.
- Get an access token: To make calls to the Power BI API, you need to get an access token. You can get the access token by using the OAuth2.0 authentication flow. There are different ways to implement OAuth2.0 in Laravel, you can use a package like “laravel/passport” or “socialite” to implement OAuth2.0 authentication.
- Create a Power BI report: To create a Power BI report, you can use Power BI Desktop or the Power BI service. Once you have created a report, publish it to the Power BI service.
- Get the report ID: To get the report ID, open the Power BI service, navigate to the report that you want to embed, and copy the report ID from the URL.
- Embed the report: To embed the report in your Laravel application, you can use the Power BI REST API. The API provides different ways to embed a report, such as using an iframe or a JavaScript SDK. You can use the “Embedding for your customers” feature to embed reports securely in your application.
Here’s an example of how you can embed a Power BI report in Laravel using the Power BI REST API:
- First, you need to register an application in the Azure portal to get the required client ID and client secret. You can follow the steps in the official Microsoft documentation to register an application: https://docs.microsoft.com/en-us/power-bi/developer/embedded/register-app
- Next, you need to authenticate the user and get an access token. You can use the Azure Active Directory Authentication Library (ADAL) for PHP to handle the authentication process. Here’s an example of how to authenticate the user and get an access token:
PHP:
use Microsoft\Azure\Adal\AuthenticationContext;
use Microsoft\Azure\Adal\ClientCredential;
$authority = 'https://login.microsoftonline.com/<your-tenant-id>';
$client_id = '<your-client-id>';
$client_secret = '<your-client-secret>';
$resource = 'https://analysis.windows.net/powerbi/api';
$authContext = new AuthenticationContext($authority);
$token = $authContext->acquireTokenForClient($resource, new ClientCredential($client_id, $client_secret));
$access_token = $token->getAccessToken();
Once you have the access token, you can use the Power BI REST API to get the embed URL and access token for the report. Here’s an example of how to get the embed URL and access token:
PHP:
$report_id = '<your-report-id>';
$api_url = 'https://api.powerbi.com/v1.0/myorg/reports/' . $report_id;
$headers = [
'Authorization: Bearer ' . $access_token,
'Content-Type: application/json'
];
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, $api_url);
curl_setopt($ch, CURLOPT_HTTPHEADER, $headers);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, 1);
$response = curl_exec($ch);
curl_close($ch);
$data = json_decode($response);
$embed_url = $data->embedUrl;
$embed_token = $data->embedToken->token;
Finally, you can embed the Power BI report in your Laravel view using the Power BI JavaScript SDK. Here’s an example of how to embed the report:
HTML:
<div id="reportContainer"></div>
<script src="https://microsoft.github.io/PowerBI-JavaScript/demo/bower_components/jquery/dist/jquery.min.js"></script>
<script src="https://microsoft.github.io/PowerBI-JavaScript/demo/bower_components/underscore/underscore-min.js"></script>
<script src="https://microsoft.github.io/PowerBI-JavaScript/demo/bower_components/powerbi-client/dist/powerbi.js"></script>
<script>
var embedConfiguration = {
type: 'report',
id: '<your-report-id>',
embedUrl: '<?php echo $embed_url; ?>',
accessToken: '<?php echo $embed_token; ?>',
settings: {
filterPaneEnabled: false,
navContentPaneEnabled: false
}
};
var $reportContainer = $('#reportContainer');
var report = powerbi.embed($reportContainer.get(0), embedConfiguration);
</script>
Note that you’ll need to replace <your-tenant-id>
, <your-client-id>
, <your-client-secret>
, and <your-report-id>
with your own values.
DreamzTech Solutions is a leading Laravel Development Company in USA with development offices in USA, UK & India. Hire Laravel Developer from our 250+ Development Team and Create your web application result-oriented, scalable, robust, secure.